반응형
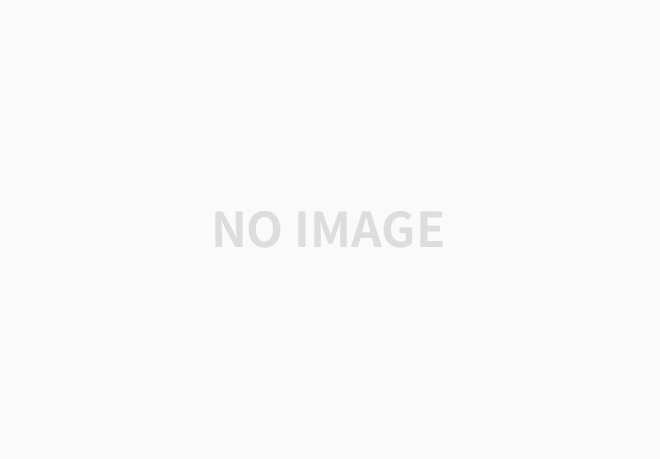
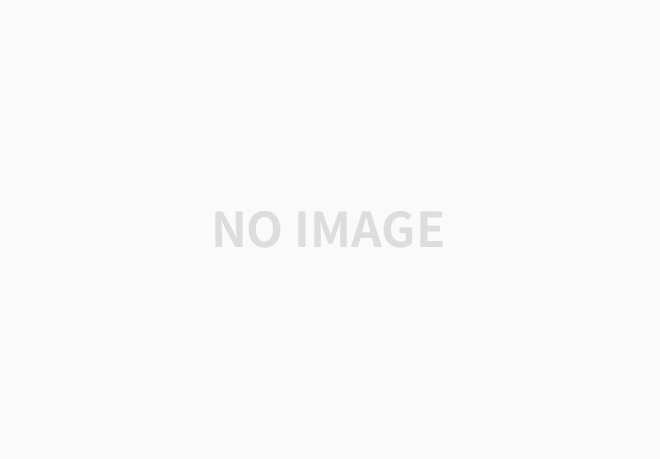
2020 카카오 인턴 2번 문제였다.
수식의 연산자에 대해 우선순위를 부여하고 그순간에서의 최대값을 구하는 문제였다. 주어진 연산자가 3개였으므로 순열을 이용하여 모든 경우의 수를 보았다. 그리고 수식의 전부를 token화 시켜서 List의 형태로 저장하였고, 계산을 할땐 list에서 remove해주는 방식으로 해주었다. 여기서 주의할점은 for문안에서 List의 사이즈를 건들수 있는 remove연산을 해주었기 때문에 index값을 조정해주는 것이 꼭필요했다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
|
package programmers;
import java.util.ArrayList;
import java.util.List;
import java.util.StringTokenizer;
public class Solution_2020카카오인턴_수식최대화 {
static String[] flags = {"*","+","-"};
static List<String> exp;
static List<String> temp;
static String[] pick;
static boolean[] visit;
static long answer;
public static void main(String[] args) {
String expression="100-200*300-500+20";
System.out.println(solution(expression));
}
static long solution(String expression) {
StringTokenizer st1 = new StringTokenizer(expression,"*-+");
StringTokenizer st2 = new StringTokenizer(expression,"0123456789");
exp=new ArrayList<>();
exp.add(st1.nextToken());
while(st1.hasMoreTokens()) {
exp.add(st2.nextToken());
exp.add(st1.nextToken());
}
temp=new ArrayList<>();
pick=new String[3];
visit=new boolean[3];
answer=0;
perm(0);
return answer;
}
static void perm(int depth) {
if(depth==3) {
copy();
calc();
return;
}
for(int i=0;i<3;i++) {
if(visit[i]==false) {
visit[i]=true;
pick[depth]=flags[i];
perm(depth+1);
visit[i]=false;
}
}
}
static void calc() {
for(int i=0;i<3;i++) {
String flag=pick[i];
for(int len=0;len<temp.size();len++) {
if(temp.get(len).equals(flag)) {
if(flag.equals("*")) {
temp.set(len, (Long.parseLong(temp.get(len-1))*Long.parseLong(temp.get(len+1))) +"");
temp.remove(len+1);
temp.remove(len-1);
}else if(flag.equals("-")) {
temp.set(len, (Long.parseLong(temp.get(len-1))-Long.parseLong(temp.get(len+1))) +"");
temp.remove(len+1);
temp.remove(len-1);
}else if(flag.equals("+")) {
temp.set(len, (Long.parseLong(temp.get(len-1))+Long.parseLong(temp.get(len+1))) +"");
temp.remove(len+1);
temp.remove(len-1);
}
len=len-2;
}
}
}
answer=Math.max(answer, Math.abs(Long.parseLong(temp.get(0))));
}
static void copy() {
temp.clear();
for(int i=0;i<exp.size();i++) {
temp.add(exp.get(i));
}
}
}
|
반응형
'Algorithm' 카테고리의 다른 글
[Programmers] 경주로 건설 (0) | 2020.08.31 |
---|---|
[Programmers] 보석 쇼핑 (0) | 2020.08.31 |
[BOJ] 1516. 게임 개발 (0) | 2020.08.30 |
[BOJ] 2668. 숫자고르기 (0) | 2020.08.29 |
[BOJ] 2294. 동전 2 (0) | 2020.08.29 |