반응형
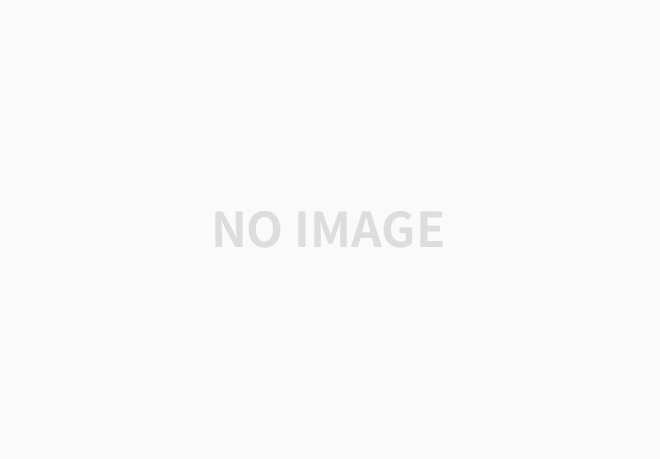
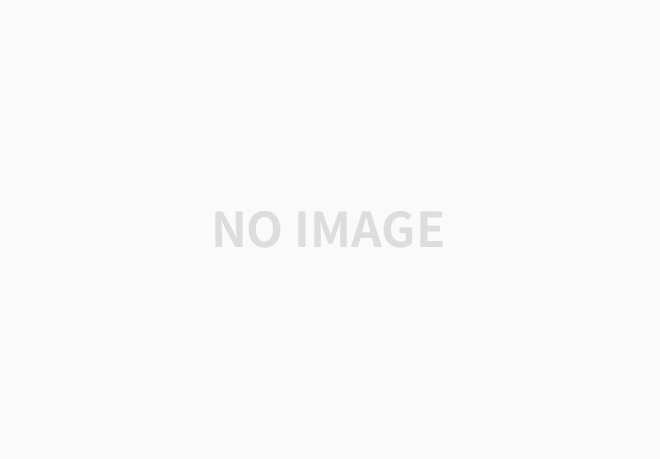
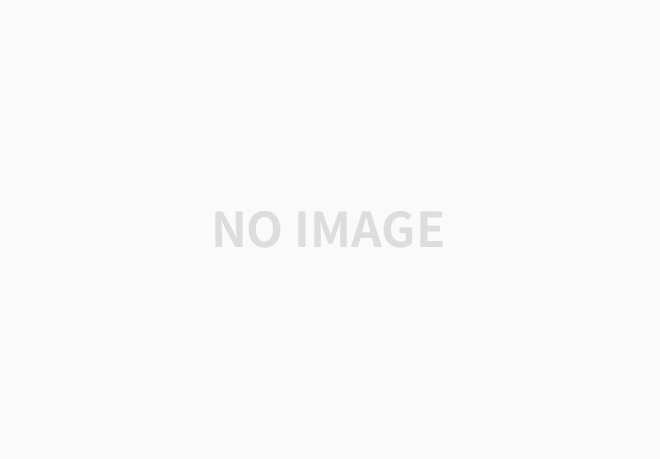
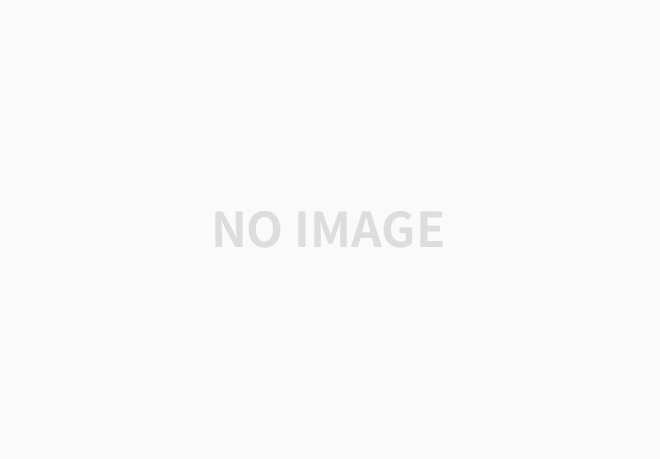
BFS를 사용하는 문제이다.
해당 칸의 값이 0이면 8칸의 값을 무조건 방문해줘야하기에 전체 배열을 돌면서 0인 곳을 찾아서 방문해주는 BFS 로직을 우선 사용하였다. 그리고 모든 방문이 끝난후 아직 방문하지 않고, 빈칸인 경우들의 대해서 count를 추가해주는 과정을 거쳐 답을 구하였다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
|
package swea;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.util.LinkedList;
import java.util.Queue;
public class Solution_d4_1868_파핑파핑지뢰찾기 {
static int[] di = {-1,-1,-1,0,1,1,1,0};
static int[] dj= {-1,0,1,1,1,0,-1,-1};
static char[][] map;
static boolean[][] visit;
static int N;
static int count=0;
static Queue<Pos> queue;
static class Pos{
int i,j;
public Pos(int i,int j) {
this.i=i;this.j=j;
}
}
public static void main(String[] args) throws Exception {
BufferedReader br =new BufferedReader(new InputStreamReader(System.in));
int T=Integer.parseInt(br.readLine());
for(int tc=1;tc<=T;tc++) {
N=Integer.parseInt(br.readLine());
map=new char[N][N];
visit= new boolean[N][N];
queue=new LinkedList<>();
for(int i=0;i<N;i++) {
String str=br.readLine();
for(int j=0;j<N;j++) {
map[i][j]=str.charAt(j);
}
}
count=0;
bfs();
System.out.println("#"+tc+" "+count);
}
}
static boolean isZero(int i,int j) {
boolean zero=true;
for(int dir=0;dir<8;dir++) {
int nextI= i+di[dir];
int nextJ= j+dj[dir];
if(nextI<0 || nextI>=N || nextJ<0 || nextJ>=N)
continue;
if(map[nextI][nextJ]=='*') {
zero=false;
break;
}
}
return zero;
}
static void bfs() {
for(int i=0;i<N;i++) {
for(int j=0;j<N;j++) {
if(map[i][j]=='.' && visit[i][j]==false && isZero(i, j)==true) {
queue.offer(new Pos(i, j));
visit[i][j]=true;
count++;
// System.out.println(i+","+j);
while(!queue.isEmpty()) {
Pos cur =queue.poll();
for(int dir=0;dir<8;dir++) {
int nextI= cur.i+di[dir];
int nextJ= cur.j+dj[dir];
if(nextI<0 || nextI>=N || nextJ<0 || nextJ>=N)
continue;
if(map[nextI][nextJ]=='.' && visit[nextI][nextJ]==false) {
if(isZero(nextI, nextJ)) {
queue.add(new Pos(nextI, nextJ));
visit[nextI][nextJ]=true;
}else
visit[nextI][nextJ]=true;
}
}
}
}
}
}
for(int i=0;i<N;i++) {
for(int j=0;j<N;j++) {
if(map[i][j]=='.' && visit[i][j]==false) {
count++;
}
}
}
}
}
http://colorscripter.com/info#e" target="_blank" style="color:#4f4f4ftext-decoration:none">Colored by Color Scripter
|
반응형
'Algorithm' 카테고리의 다른 글
[SWEA] 5656. 벽돌 깨기 (0) | 2020.03.24 |
---|---|
[SWEA] 4012. 요리사 (0) | 2020.03.24 |
[BOJ] 2447. 별 찍기-10 (0) | 2020.03.23 |
[BOJ] 2448. 별 찍기-11 (0) | 2020.03.23 |
[BOJ] 1074. Z (0) | 2020.03.22 |